imgflip.py¶
Create memes with imgflip easily!
Installation¶
To install, do
# Linux/macOS
python3 -m pip install -U imgflip.py
# Windows
py -3 -m pip install -U imgflip.py
Examples¶
CLI¶
py -3 -m imgflip -u USERNAME -p PASSWORD -tname "drake hotline bling" -top "interacting with raw imgflip api" -bot "using imgflip.py"
# use python3 on Linux/macOS
Result:
meme created!
You can find it at: https://imgflip.com/i/5gmxqp
Image link: https://i.imgflip.com/5gmxqp.jpg
Code¶
Sync
import imgflip
import requests
imgflip_client = imgflip.Imgflip(username="username", password="password", session=requests.Session()) # create an Imgflip instance
templates = imgflip_client.popular_memes(limit=10) # get popular meme templates from imgflip
meme = imgflip_client.make_meme(
template = templates["Drake Hotline Bling"],
top_text = "interacting with raw imgflip api",
bottom_text = "using imgflip.py"
) # create a meme
print(meme.url) # print the meme image url
Async
import imgflip
import aiohttp
import asyncio
async def main():
async with aiohttp.ClientSession() as session:
imgflip_client = imgflip.Imgflip(username="username", password="password", session=session) # create an Imgflip instance
templates = await imgflip_client.popular_memes(limit=10) # get popular meme templates from imgflip
meme = await imgflip_client.make_meme(
template = templates["Drake Hotline Bling"],
top_text = "interacting with raw imgflip api",
bottom_text = "using imgflip.py"
) # create a meme
print(meme.url) # print the meme image url
asyncio.run(main())
Result:
https://i.imgflip.com/5f7zzm.jpg
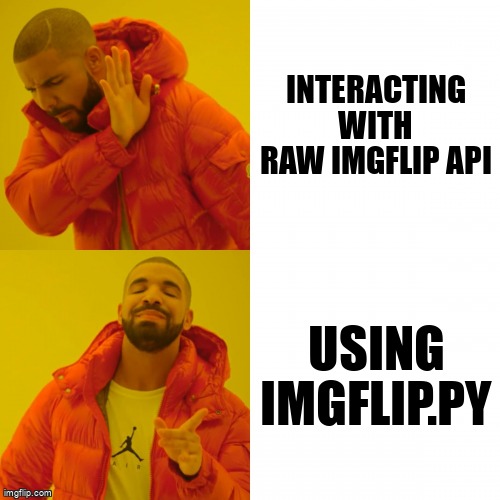